Out of the box, DonorWise supports integration with Solomon IV (4.2 and newer). If you don't use Solomon, but you'd still like to deeply integrate DonorWise with your accounting system, you can. DonorWise supports a plug-in model that supports this. Attached is a Visual Studio 2008 C# project that demonstrates this: 6685.DonorWiseGLPlugin.zip
I'll walk you through the steps it took to develop this project, as well as describe what's left for you to implement.
First of all, be sure that you're running Visual Studio 2008 "As Administrator". This is because you'll compile to the "C:\Program Files\DonorWise\plugins" folder. This folder doesn't allow write access to normal users.
When DonorWise starts up, it looks in the "plugins" folder for any .NET assemblies with any classes that implement the IDonorWiseGeneralLedgerPlugin interface. For any that exist, new entries are added to the list of "Integrated General Ledgers" available in DonorWise.
Start off by creating a new C# Class LIbrary:
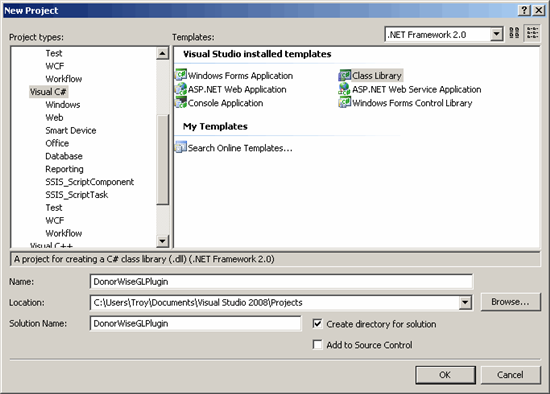
Then, add a reference to "C:\Program Files\DonorWise\DonorWise.SDK.dll" to your project:
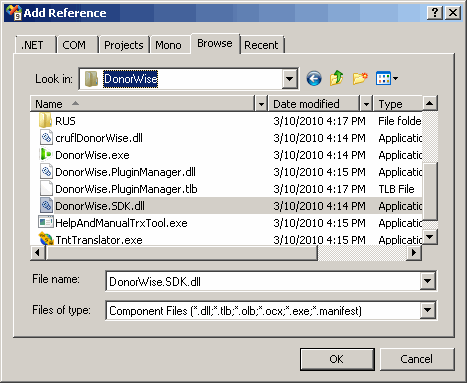
Be sure to set "Copy Local" to false in the properties window.
Under the "Project" menu, click on "Properties" and on the Build tab, set the output path to the "plugins" folder.
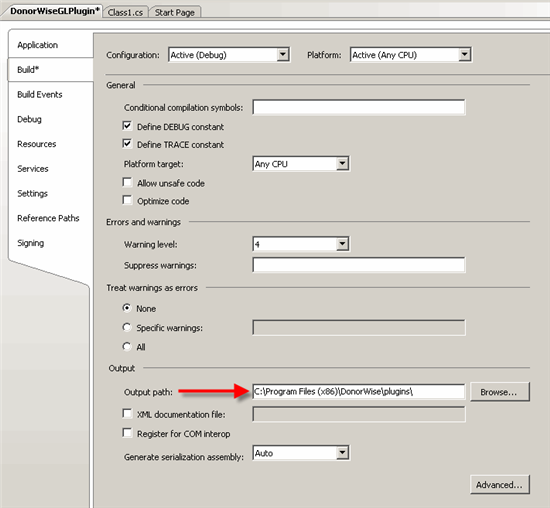
On the "Debug" tab, set it to "Start External Program" and tell it to use DonorWise.exe.
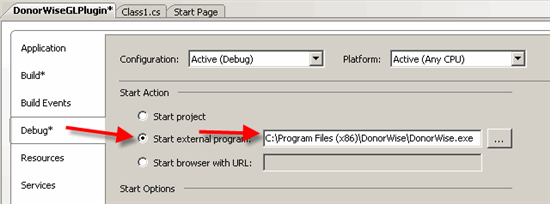
In the "Class1.cs" file, add "System.ComponentModel" and "TntWare.DonorWise.SDK" to the using list. Rename "Class1" to a more meaningful name (this shouldn't change in the future), and have it implement IDonorWiseGeneralLedgerPlugin. Add the Description attribute so it has a more user friendly name that appears in DonorWise (otherwise the class name will appear).
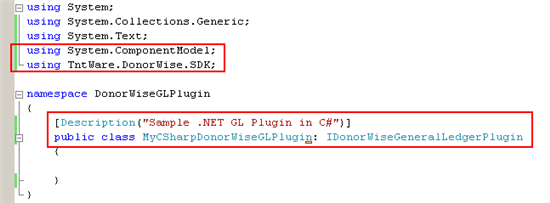
Right-click on the interface name and tell Visual Studio to implement the interface.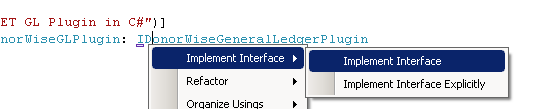
Add a reference to "System.Windows.Forms", and under the "Project" menu, click on "Add New Item". Add a Windows Form, and call it "ConfigurationForm.cs".
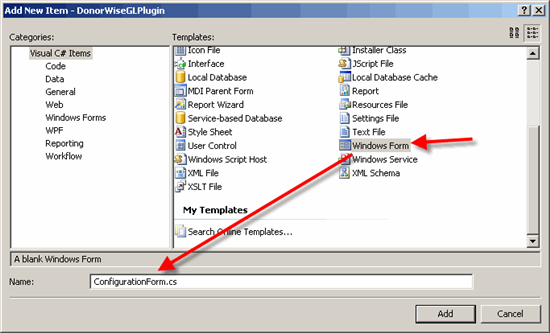
Add a check box and 2 buttons. Name the check box "EnabledCheckBox" and make sure the DialogResult for the "OK" and "Cancel" button is set appropriately.
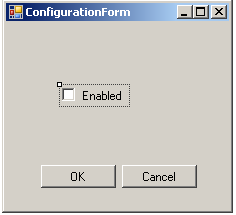
Add a public bool property "IsEnabledBox" to the form.
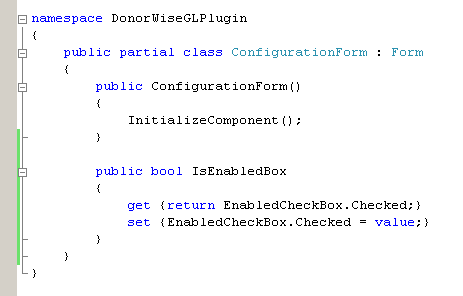
If you run the project now (F5), you'll start up DonorWise. In DonorWise, under the "Tools" menu, click on "Integrated General Ledger Options". In the list, you'll see the "Sample .NET GL Plugin in C#" we just developed. (It won't do anything because all the methods that were implemented raise an exception.)
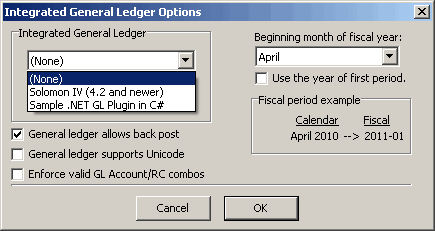
Now, I'll describe each of the methods you need to implement in the main plugin class.
-------------------------------------------------------------------------------------------------------------------------------
private bool isEnabled = false;
public string ConfigurationString
{
get
{
if (isEnabled)
return "ENABLED";
else
return "";
}
set
{
if (string.IsNullOrEmpty(value))
isEnabled = false;
else
isEnabled = true;
}
}
ConfigurationString is a property that allows DonorWise to save any configuration settings your plugin might provide. DonorWise will save the ConfigurationString when you configure the plugin, and it will load the ConfigurationString every time the program starts up. You can put whatever you want into this string. This simple example is just proof of concept.
-------------------------------------------------------------------------------------------------------------------------------
public bool Configurable()
{
return true;
}
public void PromptToConfigure()
{
ConfigurationForm frm = new ConfigurationForm();
frm.IsEnabledBox = isEnabled;
if (frm.ShowDialog() == System.Windows.Forms.DialogResult.OK)
isEnabled = frm.IsEnabledBox;
}
If your plugin provides a configuration dialog, return true for Configurable. PromptToConfigure is where you provide a user interface for the user to configure your plugin.
public void Connect()
{
if (!isEnabled)
throw new Exception("Connection not enabled.");
}
public void Disconnect()
{
//throw new NotImplementedException();
}
If your plugin uses a database connection, and you want to limit the number of times you connect, you can connect once in the Connect() method, and Disconnect once in the Disconncect() method. It's used to test the configuration from within DonorWise.
public bool HasCurrencyInfo()
{
return false;
}
public string GetBaseCurrency()
{
throw new NotImplementedException();
}
public void GetCurrencies(out TCurrency[] Currencies)
{
throw new NotImplementedException();
}
HasCurrencyInfo() should return true if your GL has currency information. Returning true allows you to specify the base currency, as well as enumerate the known currencies in use in the GL. This saves the user from manually configuring this in the "Manage Currencies Screen" and in the "System Setup" options.
public bool HasGLAccountInfo()
{
return false;
}
public void GetGLAccounts(out TGLAccount[] GLAccounts)
{
throw new NotImplementedException();
}
public void GetGLSubAccounts(out TGLSubAccount[] GLSubAccounts)
{
throw new NotImplementedException();
}
public void GetGLValidAccountCombinations(out TGLValidAccountCombination[] GLValidAccountCombinations)
{
throw new NotImplementedException();
}
HasGLAccountInfo() should true if you're able to enumerate the accounts and sub accounts (referred to as "Responsiblity Centers") within your GL.
public bool Overrides_EnforceValidCoaRc()
{
return false;
}
public bool Get_EnforceValidCoaRc()
{
throw new NotImplementedException();
}
Overrides_EnforceValidCoaRc() should return true if your able to query the GL as to wether or not it enforces valid coa/rc combinations. If Overrides_EnforceValidCoaRc() returns true, you must implement Get_EnforceValidCoaRc() and return true if the GL enforces valid combinations and false if it doesn't.
public bool Overrides_CalendarToFiscalPeriodDiff()
{
return false;
}
public int Get_CalendarToFiscalPeriodDiff()
{
throw new NotImplementedException();
}
Overrides_CalendarToFiscalPeriodDiff() should return true if you're able to query the GL as to how it defines the fiscal period. If it returns true, be sure to implement Get_CalendarToFiscalPeriodDiff() by returning the difference in months between the start of the fiscal period and the start of the calendar year.
public bool Overrides_FiscalPeriod()
{
return false;
}
public void Get_FiscalPeriod(out int PeriodMonth, out int PeriodYear)
{
throw new NotImplementedException();
}
Overrides_FiscalPeriod() should return true if you're able to query the GL as to the current fiscal period. If you return true, be sure to return the current fiscal year and period in Get_FiscalPeriod().
public bool Overrides_AccountingAllowsBackPost()
{
return false;
}
public bool Get_AccountingAllowsBackPost()
{
throw new NotImplementedException();
}
Overrides_AccountingAllowsBackPost() should return true if you're able to query the GL as to wether or not it allows posing of batches to closed periods. If you return true, be sure to implement Get_AccountingAllowsBackPost().
public bool Overrides_JournalSupportsUnicode()
{
return false;
}
public bool Get_JournalSupportsUnicode()
{
throw new NotImplementedException();
}
Overrides_JournalSupportsUnicode() should return true if you're able to query the GL (or if you just know) as to wether or not it supports any Unicode character in the journal descriptions. If you return true, be sure to implement Get_JournalSupportsUnicode().
public void PostJournal(int DonorWiseBatchID,
out string JournalPostCode, TJournalLineItem[] JournalInfo,
DateTime TranDate, int FiscalPeriod, int FiscalYear)
{
throw new NotImplementedException();
}
public bool IsJournalPostingConfirmed(int DonorWiseBatchID,
string JournalPostCode)
{
throw new NotImplementedException();
}
You must implement PostJournal(). This is where DonorWise submits a batch of transactions to the GL. The journal submitted doesn't necessarily have to post immediately. It might go into a holding state for some posting process performed later by the GL. You must return a JournalPostCode that identifies the journal within the GL. IsJournalPostingConfirmed() should return true when the journal is indeed posted to the GL.
public void DeleteJournal(int DonorWiseBatchID,
string JournalPostCode)
{
throw new NotImplementedException();
}
public bool IsJournalDeleted(int DonorWiseBatchID,
string JournalPostCode)
{
throw new NotImplementedException();
}
DeleteJournal() will only be called for unconfirmed journals (not yet fully posted). IsJournalDeleted() should return true if the journal doesn't exist in the GL.